코딩마을방범대
웹 소켓을 이용한 메시지 전송(클라이언트) 본문
728x90
웹 소켓을 이용한 메시지 전송(백엔드 - SpringBoot) - 이전포스트
이전엔 백엔드에서 웹소켓 기능을 처리하는 방법을 포스팅했었다.
이번엔 프론트에서 구독 및 메시지 받기 등을 진행할 것이다.
패키지 설치
> npm i soketjs-client, @stomp/stompjs --save
스크립트 파일
import SockJS from 'sockjs-client';
import StompJs from '@stomp/stompjs';
const client = new StompJs.Client({
brokerURL: 'ws://localhost:80/test',
connectHeaders: {
login: 'user',
passcode: 'password',
},
debug: function (str) {
console.log(str);
},
reconnectDelay: 5000
});
client.onConnect = (data) => {
const subscribeStomp = client.subscribe('/user/queue', (message) => {
if( message.command === "MESSAGE" ) {
// ...
}
});
};
client.onStompError = (err) => {
console.log('Broker reported error: ' + err.headers['message']);
console.log('Additional details: ' + err.body);
};
client.activate();
client.publish({
destination: '/app/messages',
body: JSON.stringify({
"name": "짱구",
"userKey": "dbkim"
}),
headers: {
priority: '9'
},
});
client.unsubscribe();
client.deactivate();
함수 | 설명 |
new StompJS.Client({conf}) | 웹소켓 클라이언트 연결 |
onConnect(data) | 웹소켓에 연결이 성공했을 때 실행되는 함수 |
onStompError(err) | 웹소켓에 연결 시 에러가 발생했을 때 실행되는 함수 |
activate() | 클라이언트를 활성화 시켜줌 |
deactivate() | 웹소켓 클라이언트 연결을 중지함 |
subscribe() | 구독과 동시에 인자의 콜백 함수를 실행함 ( 메시지를 전달 받음) |
unsubscribe() | 구독 취소 |
publish() | 클라이언트에서 서버로 메시지 전송 |
new StompJS.Client({conf})
1. brokerURL
- 백에서 설정한 Endpoint 경로
- http일 경우 ws, https일 경우 wss
2. connectHeader
- 서버에 보낼 데이터를 기입
3. debug
- 웹소켓의 진행 상태를 알려줌
- >>>는 서버로 보낸 것, <<<는 클라이언트에서 받은 것
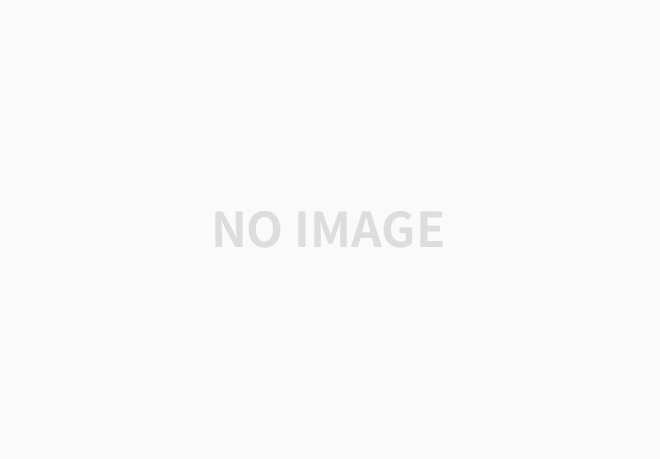
4. reconnectDelay
- 자동 재연결 시간 설정 (밀리초)
ex) 위 예제는 5초마다 자동 연결을 시도
client.publish({conf})
*은 필수항목
1. destination *
- 백에 설정되어있는 브로커 경로 설정
2. body
- 보낼 데이터 기입
( 데이터는 무조건 문자열이여야함 )
3. headers
- priority를 이용해 우선순위를 정할 수 있음
※ 실행 시 debug 콘솔로그
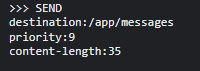
참고사이트
WebSoket (stompJS+React) 채팅 - 1
728x90
'👀 프론트엔드' 카테고리의 다른 글
React의 useRef 와 JavaScript의 객체 구조 분해 할당 (0) | 2023.07.21 |
---|---|
window에서 Node.js 여러 버전 사용하기 (0) | 2023.07.20 |
자바스크립트의 Promise 의 역할 (0) | 2023.07.20 |
Artillery를 이용해 부하 테스트 진행하기 (0) | 2023.07.18 |
프론트(web)의 package.json (0) | 2023.07.18 |